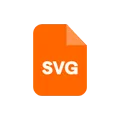
Implement and Use SVG Icons?
Implementing and using SVG (Scalable Vector Graphics) icons in your projects can significantly improve your website's or application's loading times and visual consistency across different screen sizes. SVGs are XML-based, meaning they're scalable without losing quality, making them ideal for responsive design. Here’s a detailed guide to help you implement and use SVG icons:
1. Obtaining SVG Icons
Download from Website Libraries like FontAwesomeIcons, Flaticon, or Iconmonstr offer a wide range of SVG icons that you can download and use.
2. Implementing SVG Icons in Your HTML
Embed the entire SVG code directly into your HTML where you need the icon to appear. This method allows you to treat the SVG almost like any other HTML element, making it highly customizable and interactive.
Here’s the SVG code for a simple rectangle:
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24">
<!-- Your SVG path here -->
<path d="M10 10 H 90 V 90 H 10 L 10 10"></path>
</svg>
The "d" attribute defines a path to be drawn. In this case:
- M10 10 moves the pen to start drawing at point (10,10).
- H 90 draws a horizontal line to the right until it reaches the x-coordinate 90.
- V 90 draws a vertical line downwards until it reaches the y-coordinate 90.
- H 10 draws a horizontal line back to the x-coordinate 10.
- L 10 10 closes the path by linking back to the starting point.
3. Styling Your Inline SVG Icons
Since your SVG is now part of your HTML, you can use CSS to style it. This includes changing the color, size, and even adding animations or transitions.
Changing Color:
circle {
fill: blue;
}
Changing Color on Hover:
circle:hover {
fill: red;
}
4. Manipulating SVG with JavaScript
Inline SVGs can also be manipulated with JavaScript, offering dynamic interaction capabilities. First, here's the HTML structure containing the SVG element. We'll give the SVG an id so it can be easily targeted with JavaScript.
id="mySvg"
The following JavaScript code selects the SVG element by its ID and applies a rotation animation. This is done by updating the transform property of the SVG in a loop, effectively causing it to spin.
document.addEventListener("DOMContentLoaded", function() {
var svgElement = document.getElementById('mySvg');
var angle = 0;
setInterval(function() {
angle = (angle + 2) % 360; // Increment the angle and loop back at 360
svgElement.style.transform = 'rotate(' + angle + 'deg)'; // Apply rotation
svgElement.style.transformOrigin = '50% 50%'; // Set the origin of rotation to the center
}, 20); // Update every 20 milliseconds
});
This JavaScript snippet does the following:
- Waits for the DOM to be fully loaded before executing.
- Gets the SVG element using its id.
- Sets an interval timer to update the rotation angle by 2 degrees every 20 milliseconds.
- Applies the rotation by modifying the transform property of the SVG element.
- Ensures the rotation is centered by setting the transform-origin property to the center of the element (50% 50%).
To use this JavaScript with the HTML, you can either include it directly within a "script" tag at the end of the body in your HTML document, or save it as a separate js file and reference it in your HTML.
-
Including JavaScript Directly in HTML:
Add the JavaScript code inside a tag before the closing body tag in your HTML document.
-
Referencing an External JavaScript File:
1. Add the JavaScript code inside a tag before the closing body tag in your HTML document.
2. Add the JavaScript code inside a tag before the closing body tag in your HTML document.
<script src="animation.js"></script>
5. Full Code Example
This complete example demonstrates how SVG, CSS, and JavaScript can work together to create interactive and animated web content. By manipulating SVG with CSS and JavaScript, you can create visually dynamic and engaging user experiences.
Here's how you could integrate this into a full HTML document with both CSS and javascript modifications:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>SVG, CSS, and JavaScript Example</title>
<style>
#mySvg path {
fill: lightblue; /* Default color */
transition: fill 0.3s ease; /* Smooth transition for color change */
}
#mySvg path:hover {
fill: navy; /* Color on hover */
}
</style>
</head>
<body>
<svg id="mySvg" xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24">
<!-- Your SVG path here -->
<path d="M10 10 H 90 V 90 H 10 L 10 10"></path>
</svg>
<script>
document.addEventListener("DOMContentLoaded", function() {
var svgElement = document.getElementById('mySvg');
var angle = 0;
setInterval(function() {
angle = (angle + 2) % 360; // Increment the angle and loop back at 360
svgElement.style.transform = 'rotate(' + angle + 'deg)'; // Apply rotation
svgElement.style.transformOrigin = '50% 50%'; // Set the origin of rotation to the center
}, 20); // Update every 20 milliseconds
});
</script>
</body>
</html>